LimeWire AI API Review: Seamless Content Creation for Developers
LimeWire just lately launched the AI Studio for creators and artists. Now, the corporate has launched its RESTful APIs for content material creation utilizing high generative AI fashions. Builders can leverage LimeWire’s AI API to generate pictures from textual content prompts, upscale pictures, broaden pictures past the border, and modify pictures utilizing AI.
To not point out, LimeWire can be working to deliver API assist for music and video technology as properly. So, on this article, we evaluate LimeWire AI APIs throughout many various generative AI duties. Undergo our detailed testing of the API and see if it matches your invoice.
Generate Pictures Utilizing LimeWire API
To begin with, let’s undergo the text-to-image endpoint and how one can implement the LimeWire AI API to generate pictures. I’m demonstrating the examples in Python, however you possibly can select different languages too together with Node.js, PHP, Java, Go, Ruby, and extra.
Here’s a code pattern for producing pictures in Python:
import requests
url = "https://api.limewire.com/api/picture/technology"
payload = {
"immediate": "A dinosaur on the moon",
"aspect_ratio": "1:1"
}
headers = {
"Content material-Sort": "software/json",
"X-Api-Model": "v1",
"Settle for": "software/json",
"Authorization": "Bearer "
}
response = requests.put up(url, json=payload, headers=headers)
information = response.json()
print(information)
As you possibly can see right here, I’m utilizing LimeWire’s picture technology endpoint (api/picture/technology
) and within the physique, have outlined the immediate. The immediate
ought to be lower than 2,000 characters, and it’s also possible to go a negative_prompt
to explain what shouldn’t be included within the picture. Under, I’ve specified the aspect_ratio
as 1:1
, however it’s also possible to specify 2:3
and 3:2
as properly.
Since that is the primary launch of LimeWire’s AI API, the X-Api-version
is talked about as v1
. Merely paste your LimeWire API key subsequent to Bearer
, and you might be good to go.
By the best way, there are various different parameters too which you’ll be able to embrace like samples
to generate the variety of pictures (from 1
to 4
), high quality
to specify the specified high quality (LOW
, MID
, and HIGH
, MID
is the default), fashion
to outline the picture fashion (PHOTOREALISTIC
, SCIFI
, LANDSCAPE
), steering scale to ask the mannequin to strictly adhere to your textual content immediate (from 1
to 100
, 40
is the default). You could find extra info on LimeWire’s API documentation web page.
Now, simply name the API and also you get a clear output in JSON with asset_id
and asset_url
of the picture. It’s that easy to generate pictures utilizing LimeWire’s AI API. Presently, LimeWire helps over 10 Generative AI fashions together with BlueWillow (v3, v4, and v5), Dall -E3, Steady Diffusion XL v1.0, Google AI Imagen, and extra.
Upscale Pictures Utilizing LimeWire API
Subsequent, we’ve got the API to upscale pictures utilizing LimWire’s api/picture/upscaling
endpoint. Right here, it’s worthwhile to outline the image_asset_id
which you’ll be able to generate utilizing its “api/add
” endpoint by way of a POST request, as described beneath. After that, merely point out the upscale_factor
(2
, 3
, or 4
) and paste your API key.
import requests
url = "https://api.limewire.com/api/picture/upscaling"
payload = {
"image_asset_id": "XXXXXXXXXXXXXXXXXXXXXXXXX",
"upscale_factor": 2
}
headers = {
"Content material-Sort": "software/json",
"X-Api-Model": "v1",
"Settle for": "software/json",
"Authorization": "Bearer "
}
response = requests.put up(url, json=payload, headers=headers)
information = response.json()
print(information)
It’ll return the generated asset_id
together with the asset_url
. In my testing, the LimWire API did a superb job. I handed a 1000×750 PNG picture and it upscaled the picture and generated a 2000×1500 picture with no artifacts.
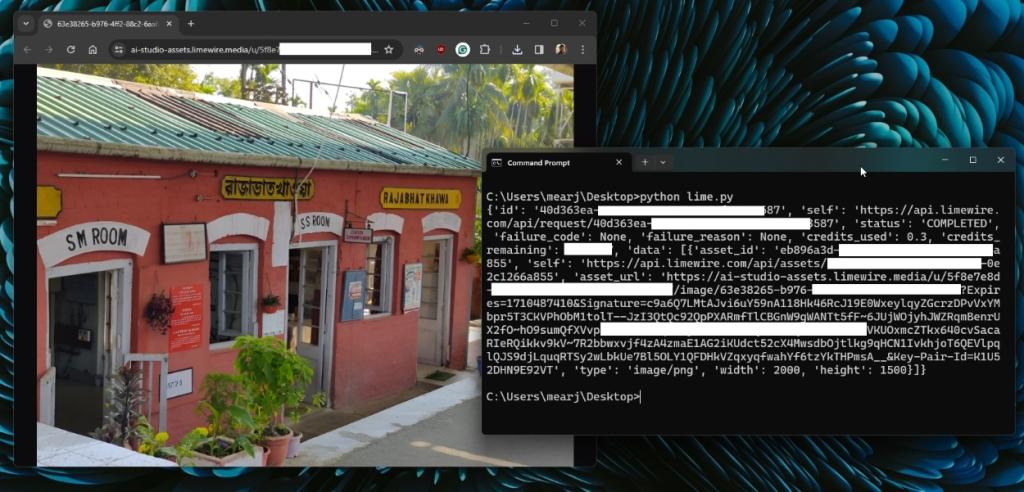
It’s typically seen that once you go a picture with texts, Diffusion fashions wrestle with texts and render them incorrectly. Nevertheless, LimeWire’s picture upscaling API didn’t botch up the textual content within the picture, which is superb.
Outpaint Pictures Utilizing LimeWire API
Now, we come to outpainting pictures which lets you lengthen the boundaries of the picture. It generates objects and backgrounds in sync with the present content material of the picture. Right here, I’m utilizing LimeWire’s api/picture/outpainting
endpoint.
Similar to above, it’s important to go the image_asset_id
and add the API key. Right here, the “route
“ parameter helps you to outline the way you need to broaden the picture, only one aspect or all instructions. You’ll be able to outline route
as UP
, DOWN
, LEFT
, RIGHT
, or ALL
.
import requests url = "https://api.limewire.com/api/picture/outpainting" payload = { "image_asset_id": "XXXXXXXXXXXXXXXXXXXXXXXXXXX", "route": "UP", "crop_side": "LEFT" } headers = { "Content material-Sort": "software/json", "X-Api-Model": "v1", "Settle for": "software/json", "Authorization": "Bearer" } response = requests.put up(url, json=payload, headers=headers) information = response.json() print(information)
Subsequent, you possibly can outline the crop_side
to specify which aspect to crop for outpainting. Not all pictures require cropping, nevertheless, AI fashions can select if it’s required to make the picture look extra pure. You’ll be able to outline it as TOP
, BOTTOM
, LEFT
, or RIGHT
. Take into account, for outpainting, your picture ought to have an side ratio of 1:1
, 2:3
or 3:2
.
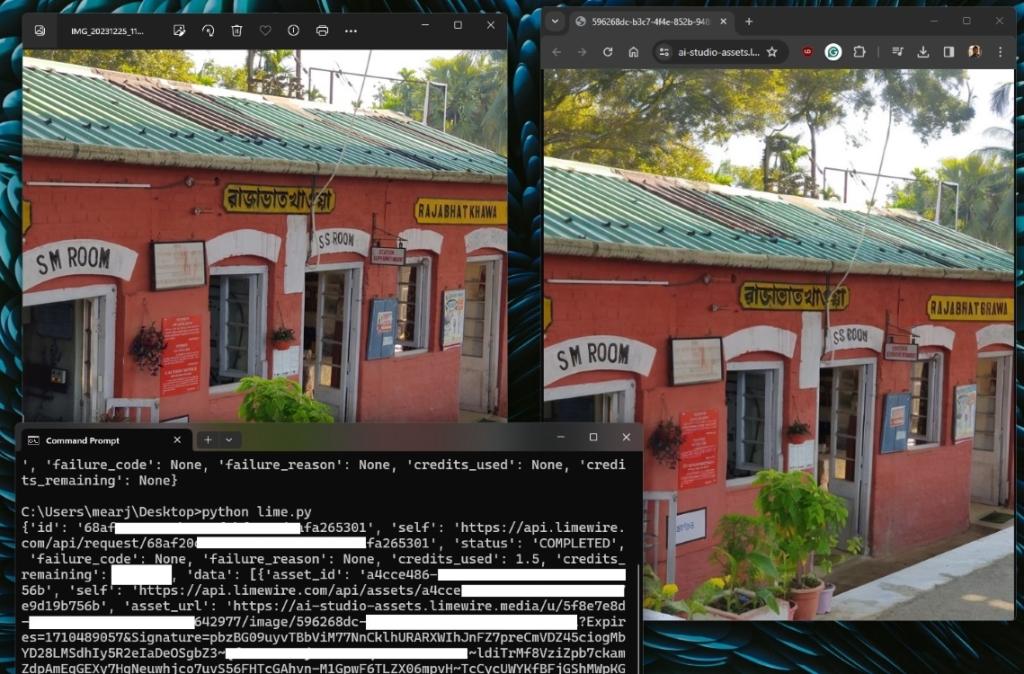
I then handed a PNG picture in 1:1
side ratio and set route
as ALL
, nevertheless it did not course of the picture. So I simply requested it to outpaint the higher border of the picture, and it efficiently processed the picture. You’ll be able to see the distinction within the above picture.
Inpaint Pictures Utilizing LimeWire API
Now, we come to inpainting which helps you to modify the picture by passing a textual content immediate. Merely add a textual content immediate together with the picture, and you possibly can manipulate the picture utilizing LimeWire’s AI API. Right here, I’m utilizing the api/picture/inpainting
endpoint.
import requests
url = "https://api.limewire.com/api/picture/inpainting"
payload = {
"image_asset_id": "XXXXXXXXXXXXXXXXXXXXXXXXX",
"immediate": "Add a tiger on the roof"
}
headers = {
"Content material-Sort": "software/json",
"X-Api-Model": "v1",
"Settle for": "software/json",
"Authorization": "Bearer "
}
response = requests.put up(url, json=payload, headers=headers)
information = response.json()
print(information)
I attempted to run the inpainting API, however sadly, it saved throwing “Inner Server Error“. The API could also be down for some purpose. However, that is how one can inpaint pictures utilizing LimeWire’s AI API.
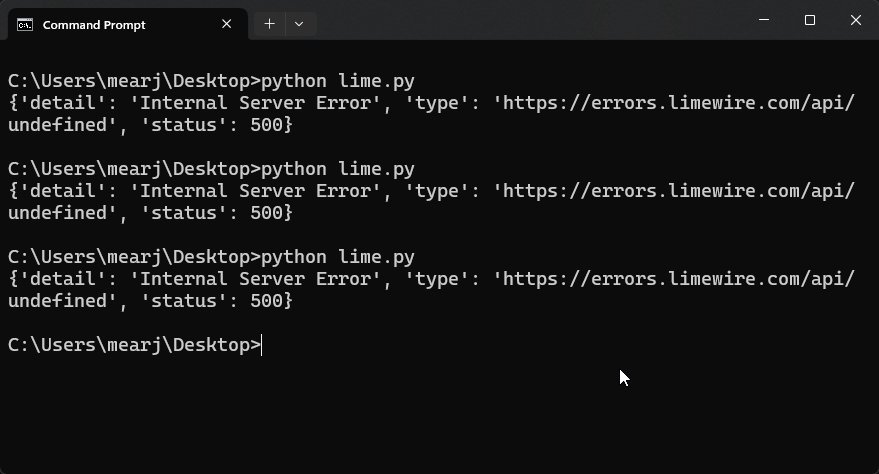
Add Property Utilizing LimeWire API
Lastly, we come to importing property utilizing LimeWire API. You should use the api/add
endpoint to add your property. LimeWire retains the uploaded property for twenty-four hours after they’re uploaded. And the most add dimension for a picture is 10MB. You should use the POST request technique to go the picture.
Be aware that, the Content material-Sort
parameter ought to match the picture format. Supported picture codecs are picture/png
, picture/jpeg
, picture/x-ms-bmp
, picture/webp
, and picture/tiff
.
import requests
url = "https://api.limewire.com/api/add"
payload = '''
[object Object]
'''
headers = {
"Content material-Sort": "picture/png",
"Authorization": "Bearer "
}
response = requests.put up(url, information=payload, headers=headers)
information = response.json()
print(information)
Is the LimeWire AI API Price It?
So far as efficiency is worried, LimeWire’s Generative API instruments are price it. The APIs are constructed on the REST structure so they’re scalable, and might ship nice efficiency. In my testing, the API calls have been resolved in 4 to five seconds, which is unimaginable. Aside from that, the standard of generated content material can be fairly good because it hosts state-of-the-art Diffusion fashions from distributors like OpenAI, Stability AI, Google, and extra.
To not point out, the API implementation is breezy and anybody can get began with the instruments in any setting. Its documentation is clear and straightforward to know. Regardless of this being the primary launch of LimeWire’s AI API, there are a number of customization choices and you’ll go a number of parameters to generate content material as you want.
As for pricing, I like what LimeWire is doing right here. It has a single plan for utilizing every kind of APIs, versus a number of plans for producing pictures, inpainting, outpainting, and so forth. that opponents are providing. The API Professional plan helps you to generate as much as 7,500 pictures per 30 days with 4 concurrent requests. It prices $49 per 30 days, which is fairly cheap compared to its opponents. For builders and small companies, this plan will serve properly.
You too can try the API Fundamental plan, which prices $29.99 per 30 days (3,750 pictures) or the API Professional Plus plan which prices $250 per 30 days (37,500 pictures).