How to Access and Use Google Gemini API Key (with Examples)
After Gemini AI’s announcement, Google has launched API entry for its Gemini fashions. Presently, the corporate is providing API entry to Gemini Professional, together with text-only and text-and-vision fashions. It’s an fascinating launch as a result of thus far, Google has not added the visible functionality to Bard as it’s operating the text-only mannequin. With this API key, you’ll be able to lastly take a look at Gemini’s multimodal functionality in your pc regionally straight away. On that notice, let’s discover ways to entry and use the Gemini API on this information.
Notice:
The Google Gemini API key’s free for now for each textual content and imaginative and prescient fashions. It will likely be free till basic availability early subsequent 12 months. So, you’ll be able to ship as much as 60 requests per minute with out having to arrange Google Cloud billing or incurring any prices.
Set Up Python and Pip on Your Laptop
- Head to our information and set up Python together with Pip in your PC or Mac. You could set up Python 3.9 or above model.
- If in case you have a Linux pc, you’ll be able to comply with our tutorial to put in Python and Pip on Ubuntu or different distros.
- You’ll be able to run the beneath instructions within the Terminal to confirm Python and Pip set up in your pc. It ought to return the model quantity.
python -V
pip -V
- As soon as the set up is profitable, run the beneath command to set up Google’s Generative AI dependency.
pip set up -q -U google-generativeai
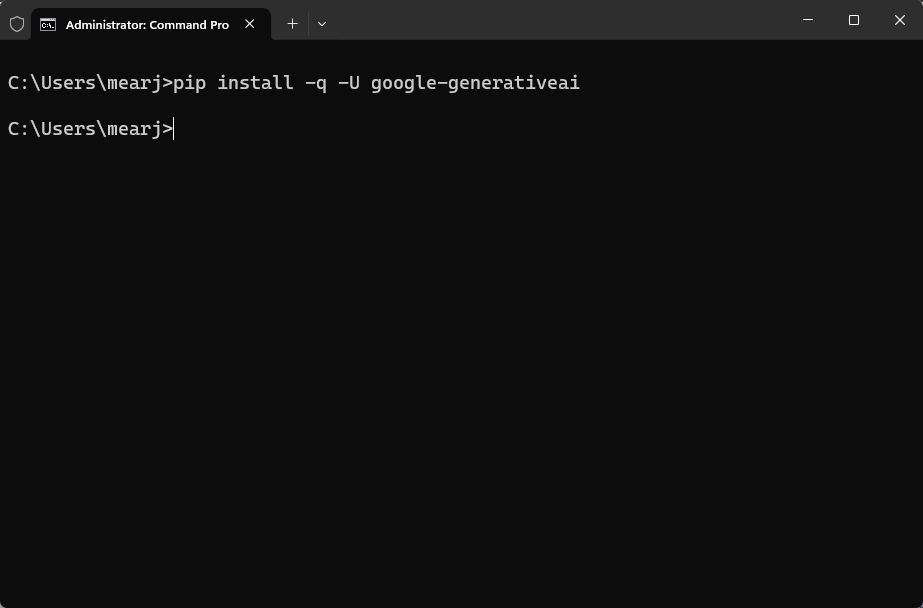
Tips on how to Get the Gemini Professional API Key
- Subsequent, head over to makersuite.google.com/app/apikey (visit) and sign up along with your Google account.
- Beneath API keys, click on the “Create API key in new undertaking” button.
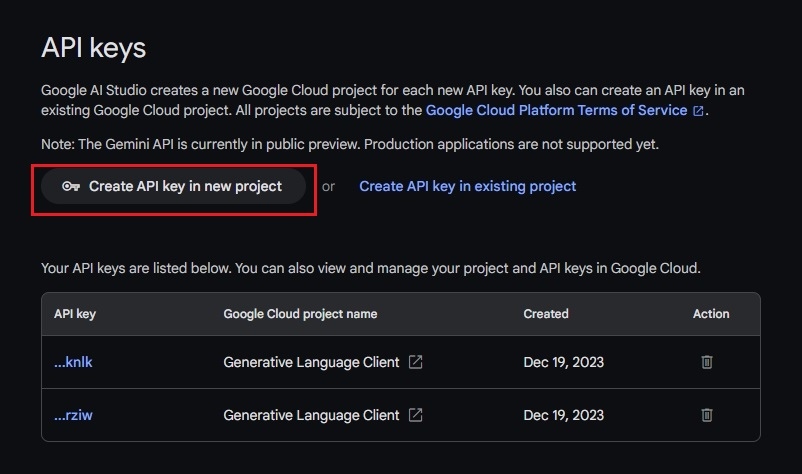
- Copy the API key and preserve it non-public. Don’t publish or share the API key publicly.
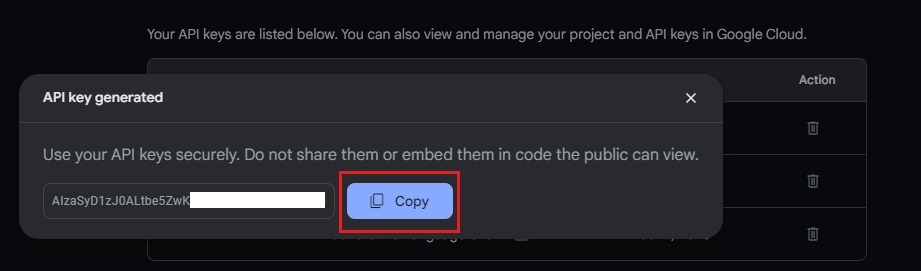
Tips on how to Use the Gemini Professional API Key (Textual content-only Mannequin)
Just like OpenAI, Google has made it easy to make use of its Gemini API key for improvement and testing functions. I’ve made the code fairly easy for the final person to check and use it. On this instance, I display how you can use the Gemini Professional Textual content mannequin by way of the API key.
- First off, launch a code editor of your selection. If you’re a newbie, merely set up Notepad++ (visit). For superior customers, Visible Studio Code (visit) is a superb device.
- Subsequent, copy the beneath code and paste it into your code editor.
import google.generativeai as genai
genai.configure(api_key='PASTE YOUR API KEY HERE')
mannequin = genai.GenerativeModel('gemini-pro')
response = mannequin.generate_content("What's the which means of life?")
print(response.textual content)
- Within the code editor, paste your Gemini API key. As you’ll be able to see, we’ve outlined the ‘gemini-pro’ mannequin, which is a text-only mannequin. Additionally, we’ve added a question the place you’ll be able to ask questions.
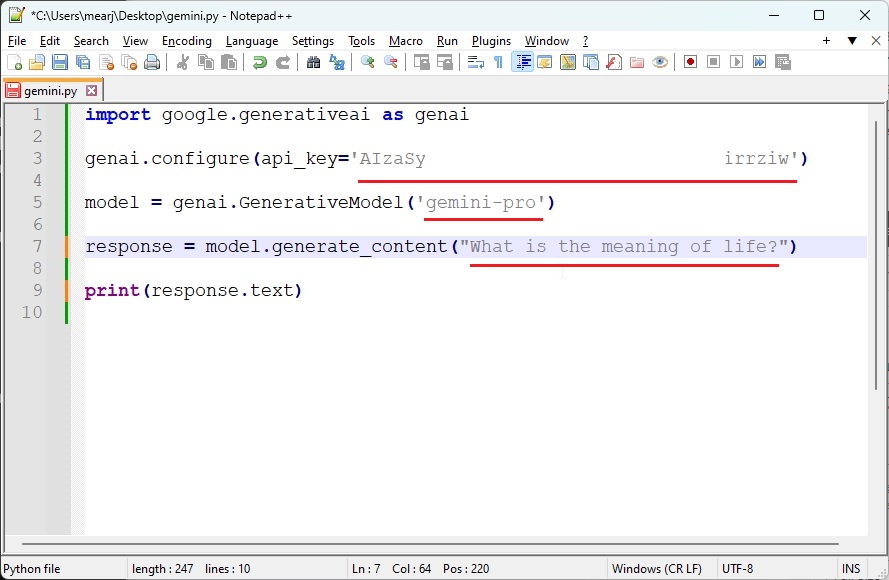
- Now, save the code and provides a reputation to the file. Make certain so as to add
.py
on the finish. I’ve named my filegemini.py
and saved it on the Desktop.
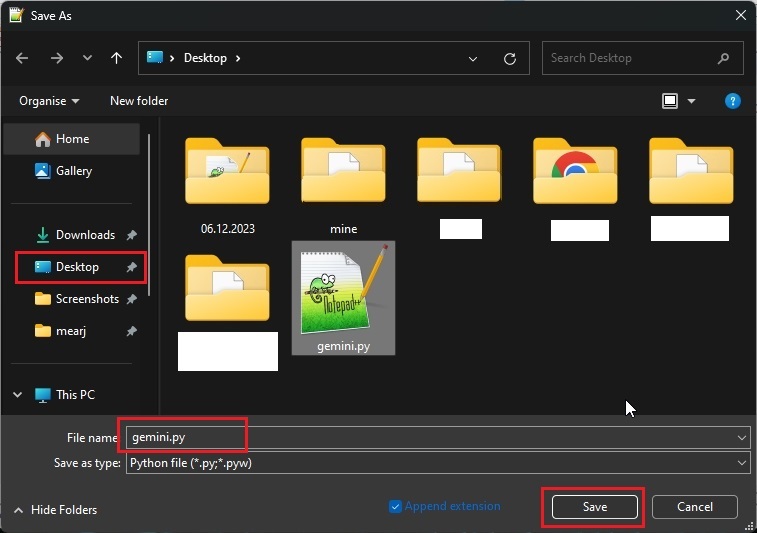
- Subsequent, hearth up the Terminal and run the beneath command to transfer to the Desktop.
cd Desktop
- As soon as you might be on the Desktop within the Terminal, merely run the beneath command to execute the
gemini.py
file utilizing Python.
python gemini.py
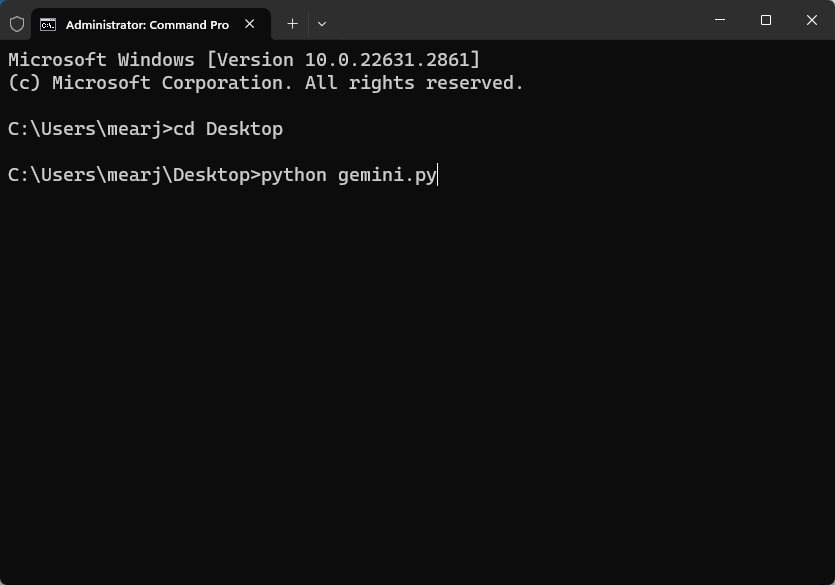
- It’ll now reply the query you had set within the
gemini.py
file.
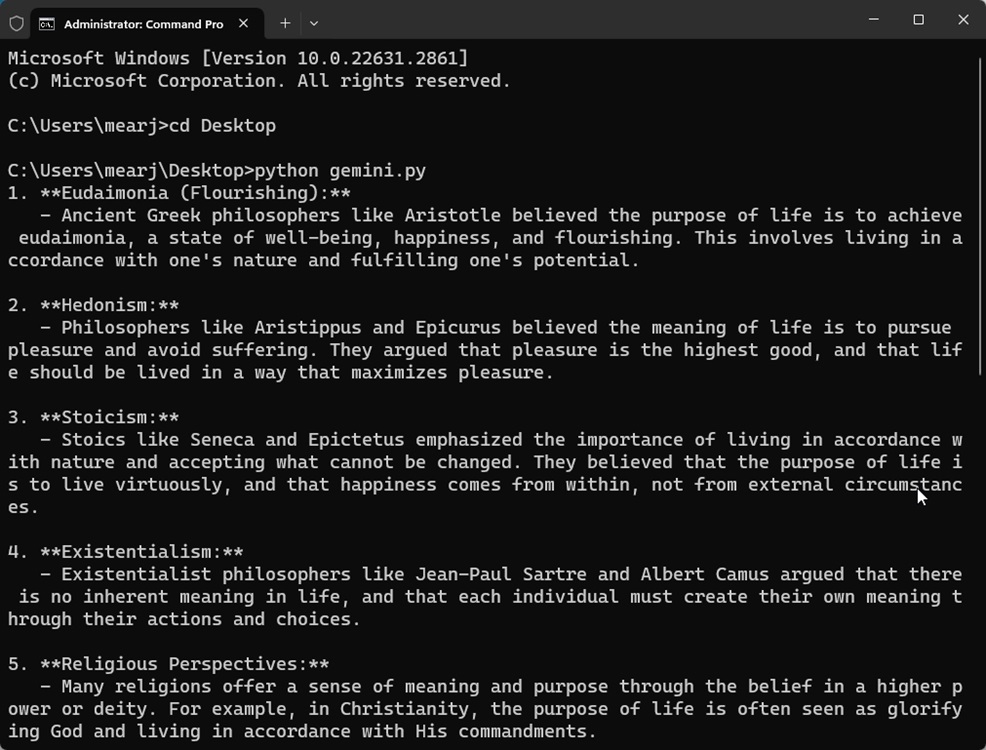
- You’ll be able to change the query within the code editor, put it aside, and run the
gemini.py
file once more to get a brand new response proper within the Terminal. That’s how you should use the Google Gemini API key to entry the text-only Gemini Professional mannequin.
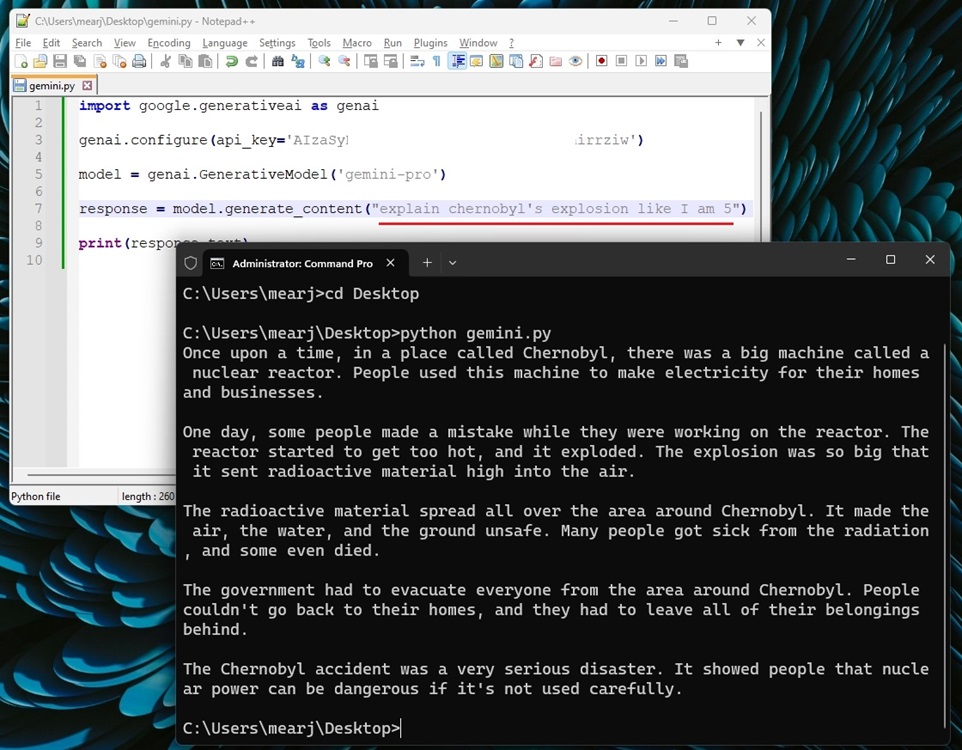
Tips on how to Use the Gemini Professional API Key (Textual content-and-Imaginative and prescient Mannequin)
On this instance, I’m going to indicate how one can work together with the Gemini Professional multimodal mannequin. It’s not stay on Google Bard but, however by way of the API, you’ll be able to entry it straight away. And fortunately, the method is once more fairly simple and seamless.
- Open a brand new file in your code editor and paste the beneath code.
import google.generativeai as genai
import PIL.Picture
img = PIL.Picture.open('picture.jpg')
genai.configure(api_key='PASTE YOUR API KEY HERE')
mannequin = genai.GenerativeModel('gemini-pro-vision')
response = mannequin.generate_content(["what is the total calorie count?", img])
print(response.textual content)
- Make certain to stick your Gemini API key. Right here, we’re utilizing the
gemini-pro-vision
mannequin, which is a text-and-vision mannequin.
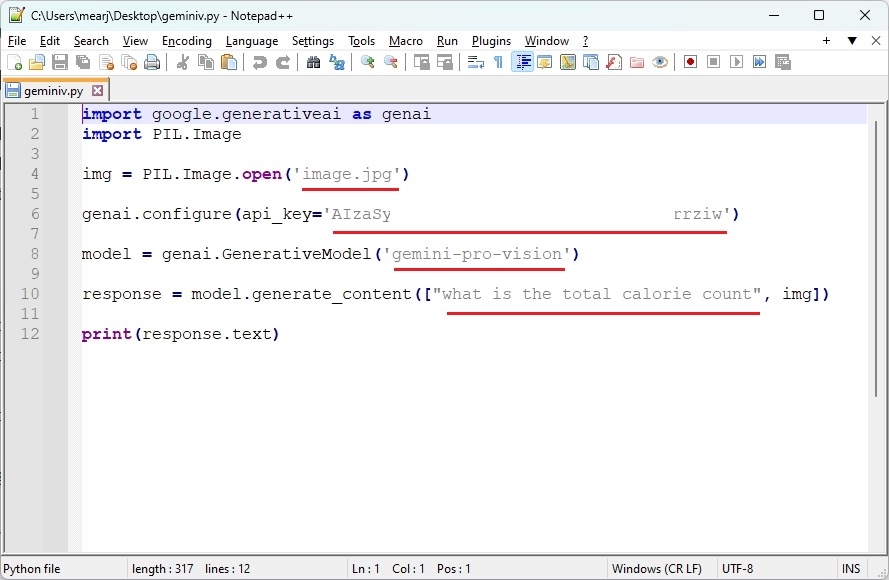
- Now, save the file in your Desktop and add
.py
on the finish of the filename. I’ve named itgeminiv.py
right here.

- Within the third line of the code, as you’ll be able to see, I level the AI to an
picture.jpg
file that’s saved on my Desktop with the precise identify. No matter picture you need to course of, make sure that it’s saved in the identical location asgeminiv.py
file, and the filename is similar with the proper extension. You’ll be able to cross native JPG and PNG recordsdata as much as 4MB.
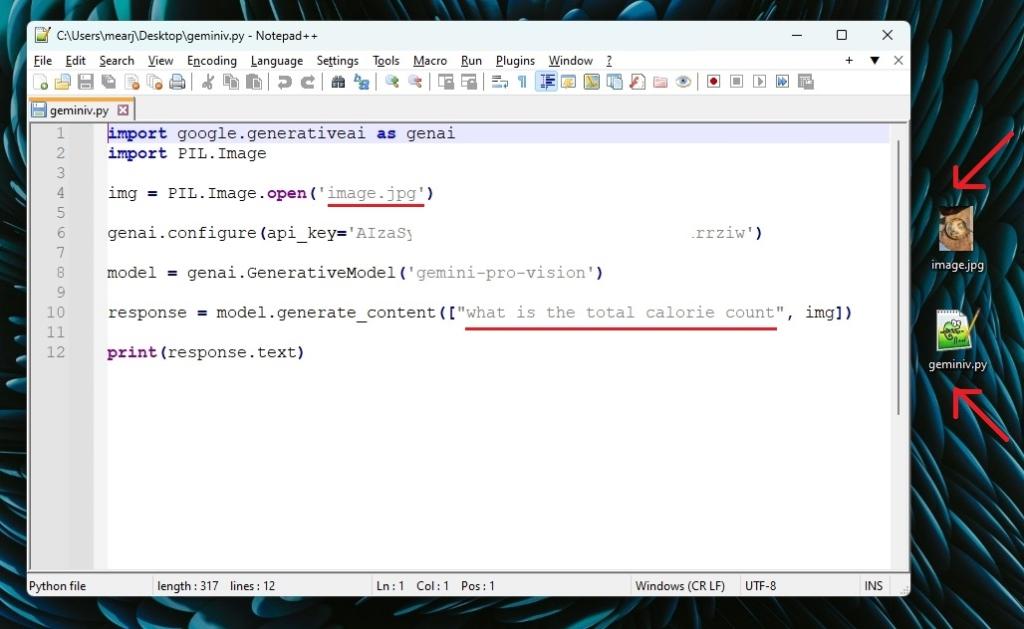
- Within the sixth line of code, you’ll be able to ask questions associated to the picture. Since I’m feeding a food-related picture, I’m asking Gemini Professional to calculate the full calorie rely.
- It’s time to run the code within the Terminal. Merely transfer to the Desktop (in my case), and run the beneath instructions one after the other. Make certain to avoid wasting the file in case you have made any modifications.
cd Desktop
python geminiv.py
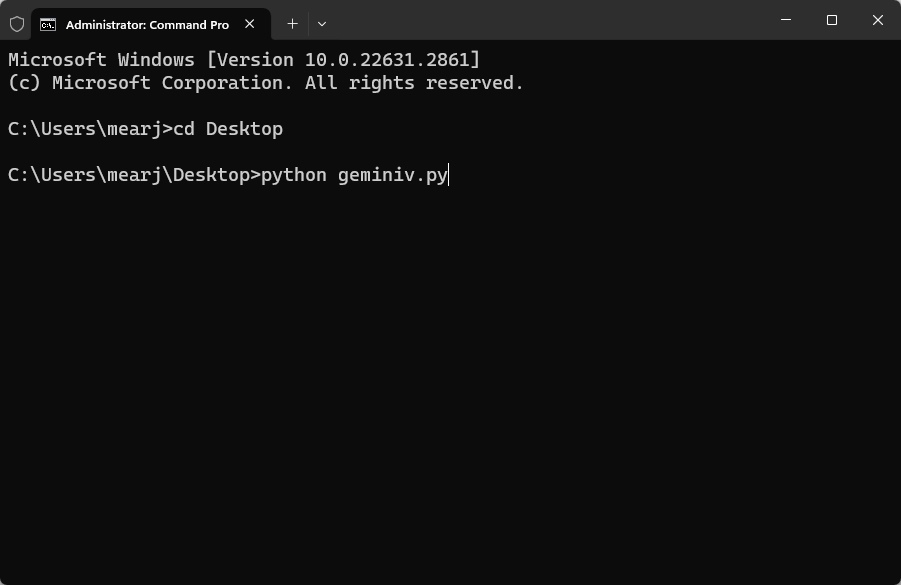
- The visible Gemini Professional mannequin solutions the query straight up. You’ll be able to ask additional questions and ask the AI to clarify the reasoning.
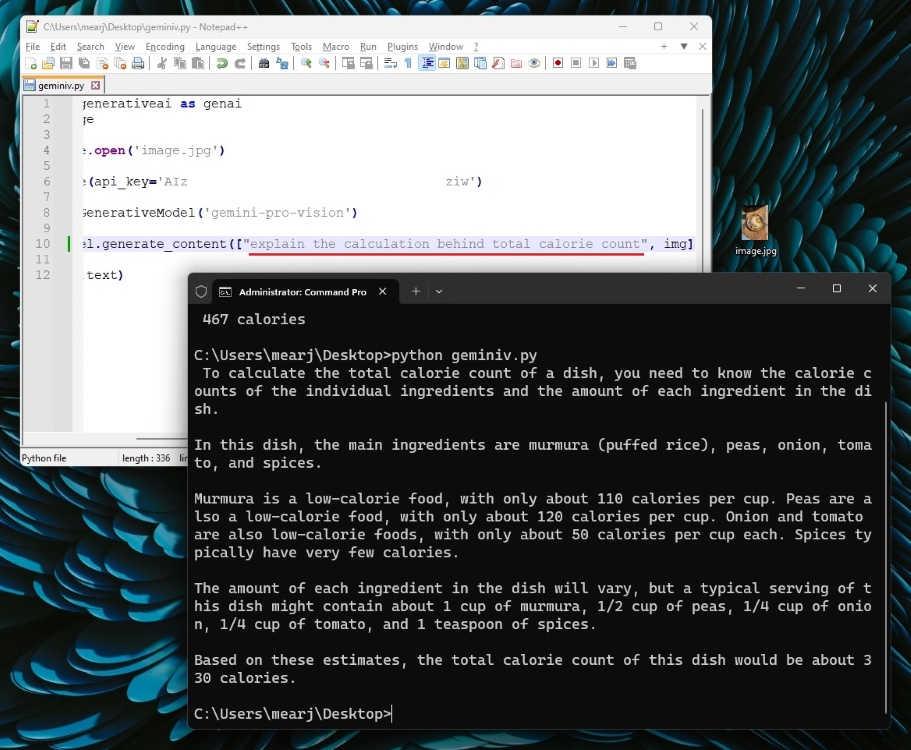
- You’ll be able to feed a special picture as properly, however make sure that to match the picture filename, change the query within the code, and run the
geminiv.py
file once more to get new responses.
Advisable Articles
Tips on how to Prepare an AI Chatbot With Customized Information Base Utilizing ChatGPT API
Mar 14, 2023
Tips on how to Use the Gemini Professional API Key in Chat Format
Due to unconv’s (GitHub) concise code, you’ll be able to chat with the Gemini Professional mannequin within the Terminal window utilizing a Gemini AI API key. This manner, you don’t have to alter the query within the code and rerun the Python file to get a brand new output. You’ll be able to proceed the chat within the Terminal window itself.
Better of all, Google has natively applied chat historical past so that you don’t have to manually append the responses and handle a chat historical past by yourself in an array or an inventory. With a easy operate, Google shops all of the dialog historical past in a chat session. Right here is the way it works.
- Open your code editor and paste the beneath code.
import google.generativeai as genai
genai.configure(api_key='PASTE YOUR API KEY HERE')
mannequin = genai.GenerativeModel('gemini-pro')
chat = mannequin.start_chat()
whereas True:
message = enter("You: ")
response = chat.send_message(message)
print("Gemini: " + response.textual content)
- As normal, paste your API key just like the above sections.
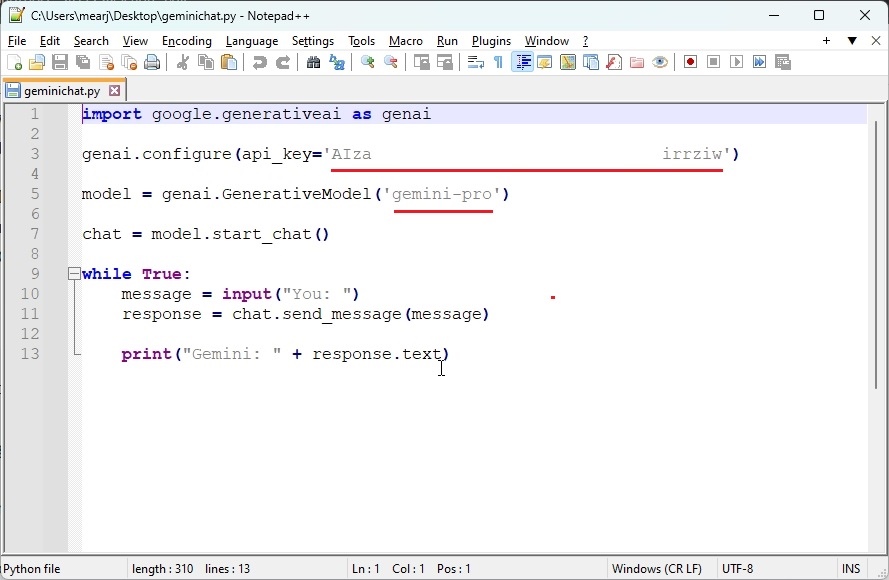
- Now, save the file on the Desktop or your most popular location. Make certain so as to add
.py
on the finish. I’ve named itgeminichat.py
file.
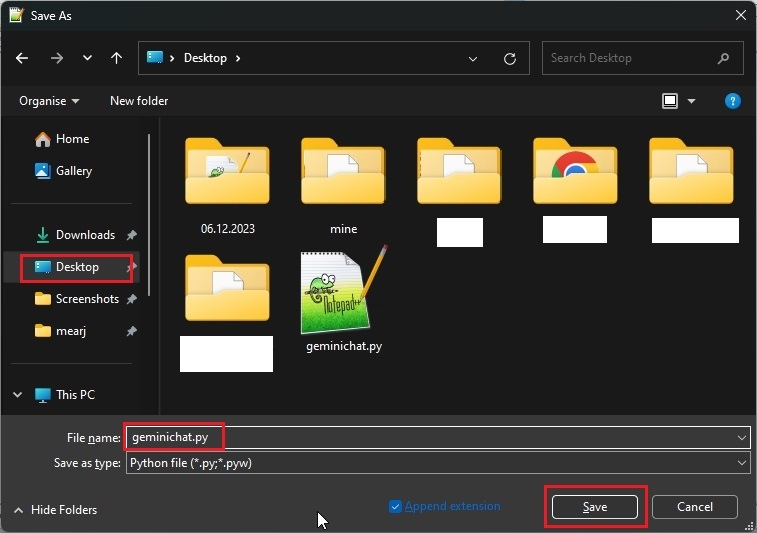
- Now, launch the Terminal and transfer to the Desktop. After that, run the
geminichat.py
file.
cd Desktop
python geminichat.py
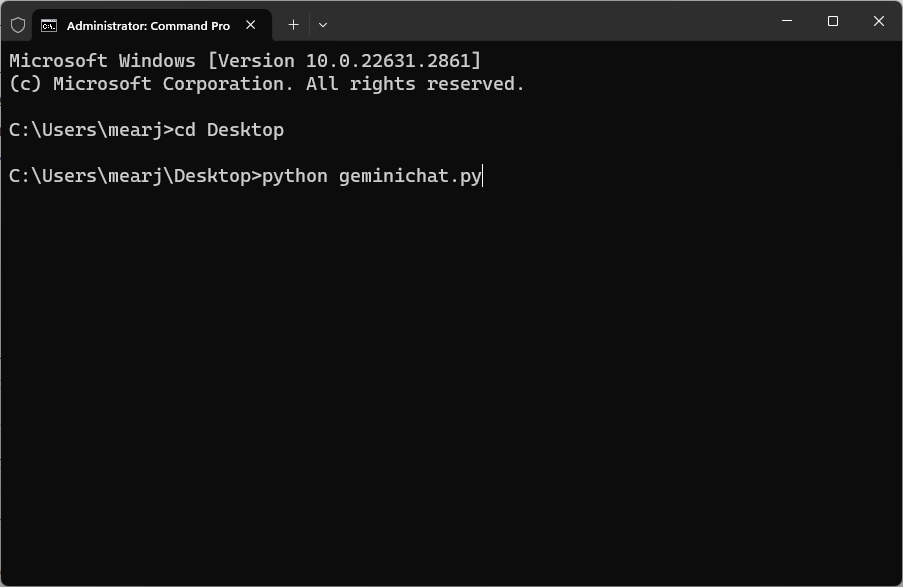
- Now you can proceed the dialog effortlessly, and it’ll additionally keep in mind chat historical past. So that is one other smart way to make use of the Google Gemini API key.
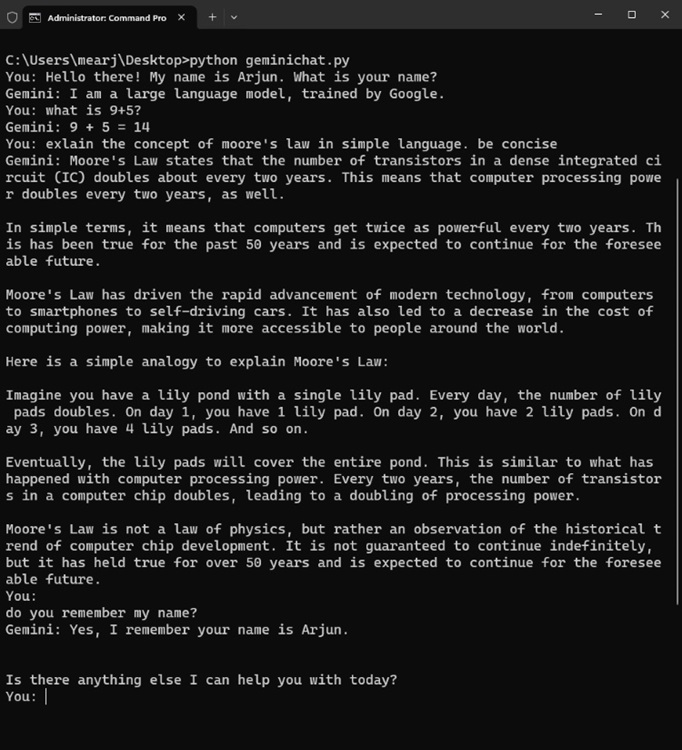
So these are a number of examples you’ll be able to strive to take a look at Google Gemini’s capabilities by way of the API. I like the truth that Google has made its imaginative and prescient mannequin accessible for fans and builders to offer it a strive, evaluating it to OpenAI’s DALL-E 3 and ChatGPT. Whereas the Gemini Professional imaginative and prescient mannequin doesn’t beat the GPT-4V mannequin, it’s fairly good nonetheless. We’re ready for the Gemini Extremely’s launch which is on par with the GPT-4 mannequin.
Aside from that, the responses from the Gemini Professional API really feel a bit totally different from Google Bard, which is additionally powered by a finetuned model of Gemini Professional. Bard’s responses appear barely boring and sanitized, however Gemini Professional’s API responses really feel extra energetic and have character.
We might be monitoring all of the modifications on this area, so keep tuned for extra Gemini AI-related content material. In the meantime, go forward and take a look at the Google Gemini API by your self.